Integrating React Native IAP into Your Expo App

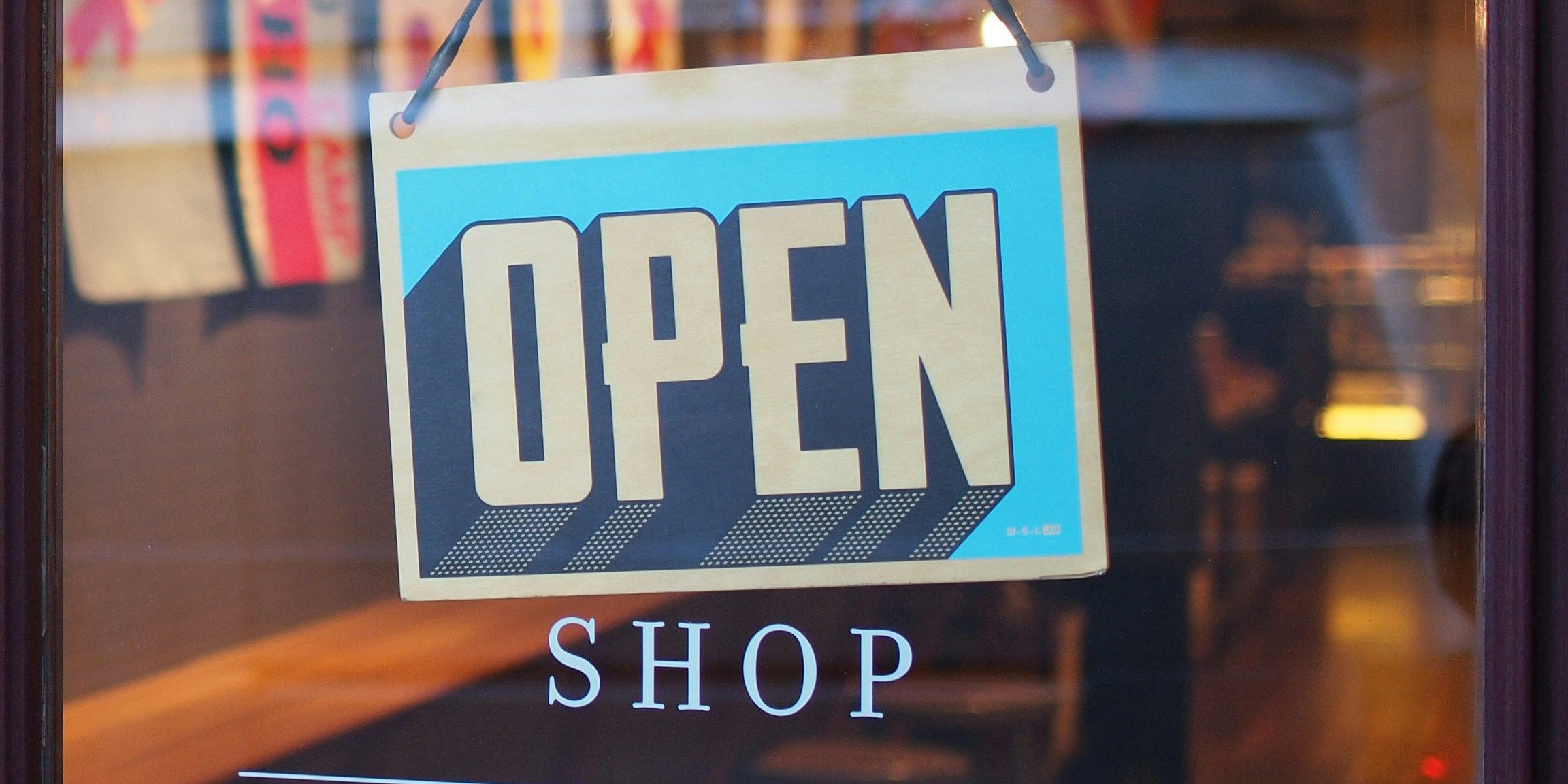

Integrating React Native IAP into Your Expo App
If you're building a mobile app with Expo and want to enable in-app purchases (IAP), you might have run into the issue that Expo doesn't natively support IAP out of the box. Luckily, you can still add in-app purchases functionality by using React Native IAP with a little configuration.
In this post, we'll walk through how to integrate React Native IAP (a popular library for in-app purchases) into your Expo-managed React Native project.
What You'll Need
- Expo SDK: You should have an Expo project already set up.
- React Native IAP: The library that allows you to handle in-app purchases on both iOS and Android.
Steps to Integrate React Native IAP with Expo
Step 1: Ejecting from Expo Managed Workflow
Unfortunately, Expo Managed Workflow does not natively support in-app purchases, so you'll need to eject your Expo project to the Bare Workflow. This step will give you access to native code, allowing you to use libraries like react-native-iap
that require native dependencies.
Run the following command to eject:
expo eject
During the eject process, Expo will prompt you with questions about your project configuration (e.g., package name, whether you want to build for iOS and Android). Follow the prompts to complete the process.
After ejecting, your project will have both an iOS and Android directory with native code, which means you now have full control over the project’s native setup.
Step 2: Install React Native IAP
Once your project is ejected, you can install the necessary packages for React Native IAP.
npm install react-native-iap
Additionally, you'll need to link the native modules to your project. Since React Native 0.60 and above supports auto-linking, this should be done automatically, but in case it doesn't, you can run:
npx react-native link react-native-iap
Step 3: Set Up Your iOS and Android Projects
For iOS, you'll need to make sure your project is set up for in-app purchases. You’ll need to enable in-app purchases in the Apple Developer Console.
- Go to the Apple Developer portal and create an App ID for your app.
- Under Capabilities, enable In-App Purchases for both the app and the target.
- You’ll also need to configure your app in App Store Connect for in-app purchase functionality. This involves setting up the products (consumables, subscriptions, etc.) that users can purchase.
For Android:
- Set up your in-app purchase items in the Google Play Console.
- Ensure the Google Play Billing Library is enabled.
Step 4: Implement In-App Purchases in Your Code
With everything set up, you can now integrate React Native IAP into your app. Below is an example of how to initiate and use in-app purchases in your app.
import React, { useEffect, useState } from 'react';
import { View, Button, Text } from 'react-native';
import RNIap from 'react-native-iap';
const itemSkus = ['com.example.yourapp.premium_item']; // SKU(s) for your in-app purchases
const InAppPurchaseScreen = () => {
const [isReady, setIsReady] = useState(false);
const [availableItems, setAvailableItems] = useState([]);
const [purchase, setPurchase] = useState(null);
useEffect(() => {
const initIAP = async () => {
try {
await RNIap.initConnection();
const products = await RNIap.getProducts(itemSkus);
setAvailableItems(products);
setIsReady(true);
} catch (err) {
console.warn('Error initializing IAP', err);
}
};
initIAP();
return () => {
RNIap.endConnection();
};
}, []);
const requestPurchase = async (sku) => {
try {
const purchase = await RNIap.requestPurchase(sku);
setPurchase(purchase);
} catch (err) {
console.warn('Error purchasing item', err);
}
};
return (
<View style={{ padding: 20 }}>
{isReady ? (
<View>
<Text>Available Products:</Text>
{availableItems.map((item) => (
<Button
key={item.productId}
title={`Buy ${item.title} for ${item.localizedPrice}`}
onPress={() => requestPurchase(item.productId)}
/>
))}
{purchase && (
<Text>
Purchase successful! {purchase.productId} - {purchase.transactionId}
</Text>
)}
</View>
) : (
<Text>Loading products...</Text>
)}
</View>
);
};
export default InAppPurchaseScreen;
In this code:
- We initialize the IAP connection with
RNIap.initConnection()
. - We retrieve available products using
RNIap.getProducts()
and display them as purchase buttons. - We handle the purchase flow with
RNIap.requestPurchase()
, and show the result once the purchase is successful.
Step 5: Handle Purchase Validation and Confirmation
After a purchase is completed, it’s important to validate and confirm the transaction. React Native IAP provides methods like RNIap.validateReceiptIOS()
for iOS and RNIap.consumePurchaseAndroid()
for Android to handle these validations.
Be sure to implement these steps for both platforms to ensure the purchase process is secure.
Step 6: Test Your In-App Purchases
Before you can start accepting real payments, be sure to thoroughly test your in-app purchases. You can use TestFlight for iOS and Google Play’s internal testing for Android.
- For iOS, you’ll need to configure sandbox testers in the App Store Connect portal.
- For Android, use the Google Play Console to test purchases using test accounts.
Step 7: Build and Publish Your App
Once everything is working as expected, you can use Expo’s Bare Workflow to build your app for production:
npx react-native run-ios
npx react-native run-android
Once everything is ready, follow the usual steps to upload your app to the App Store or Google Play.
Final Thoughts
Integrating in-app purchases in an Expo app requires ejecting to the Bare Workflow, but once that's done, React Native IAP is a reliable library for adding purchase functionality. Make sure to test thoroughly, handle transaction validation, and follow platform-specific guidelines for a smooth user experience.
Good luck with your app, and happy coding! ✨